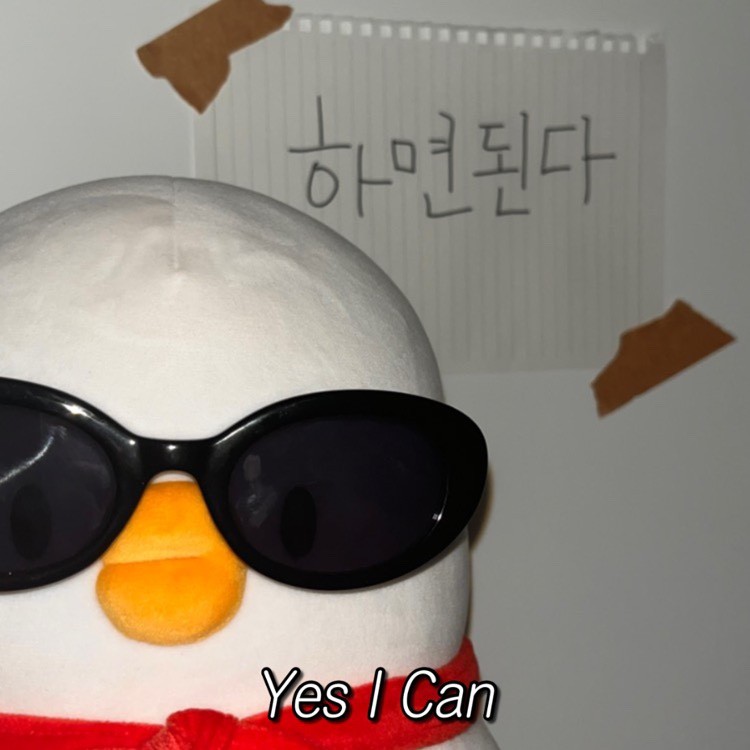
๋ผ์ด๋ธ๋ฌ๋ฆฌ ๋ถ๋ฌ์ค๊ธฐ
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
๋ฒ ์ด์ง ๋ฌธํญ
1. ๋ฐ์ดํฐ ๋ถ๋ฌ์ค๊ธฐ
pandas๋ฅผ importํ ๋ค์ ๋ฐ์ดํฐ๋ฅผ ๋ถ๋ฌ์์ ๋ฐ์ดํฐ๋ฅผ ํ์ธํ์ธ์.
# ๋ฐ์ดํฐ ๋ถ๋ฌ์ค๊ธฐ
url = 'https://archive.ics.uci.edu/ml/machine-learning-databases/iris/iris.data'
columns = ['Sepal Length', 'Sepal Width', 'Petal Length', 'Petal Width', 'Species']
iris = pd.read_csv(url, header=None, names=columns)
2. ๋ฐ์ดํฐ ๊ตฌ์กฐ ํ์ ํ๊ธฐ
๋ฐ์ดํฐ์ ์ ์ฒซ 5ํ์ ์ถ๋ ฅํ๊ณ , ๋ฐ์ดํฐ์ ๊ตฌ์กฐ๋ฅผ ํ์ ํ์ธ์.
iris.head()
- ๋ฐ์ดํฐํ๋ ์์์ ์ฒ์ 5๊ฐ์ ํ์ ์ถ๋ ฅํ๋ ํจ์ โ head()
3. ๋ฐ์ดํฐ ์์ฝ์ ๋ณด ํ์ธํ๊ธฐ
๋ฐ์ดํฐ์ ์ ์์ฝ ์ ๋ณด๋ฅผ ํ์ธํ์ธ์.
iris.info()
## <class 'pandas.core.frame.DataFrame'>
## RangeIndex: 150 entries, 0 to 149
## Data columns (total 5 columns):
## # Column Non-Null Count Dtype
## --- ------ -------------- -----
## 0 Sepal Length 150 non-null float64
## 1 Sepal Width 150 non-null float64
## 2 Petal Length 150 non-null float64
## 3 Petal Width 150 non-null float64
## 4 Species 150 non-null object
## dtypes: float64(4), object(1)
## memory usage: 6.0+ KB
- ๋ฐ์ดํฐ์ ์ ์์ฝ ์ ๋ณด๋ฅผ ํ์ธํ๋ ํจ์ โ info()
4. ๊ธฐ์ด ํต๊ณ๋ ํ์ธํ๊ธฐ
๊ฐ ์ด์ ๊ธฐ์ด ํต๊ณ๋(ํ๊ท , ํ์คํธ์ฐจ, ์ต์๊ฐ, ์ต๋๊ฐ ๋ฑ)์ ์ถ๋ ฅํ์ธ์.
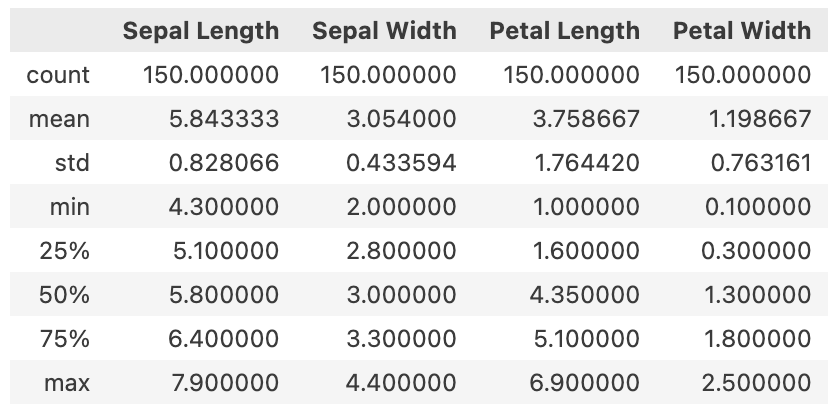
iris.describe()
- ๊ธฐ์ดํต๊ณ๋์ ์ถ๋ ฅํ๋ ํจ์ โ describe()
5. ํน์ ์ด ์ ํํ๊ธฐ
'Sepal Length' ์ด๋ง ์ ํํ์ฌ ์ถ๋ ฅํ์ธ์.
# ํ๋ค์ค ํจ์ ์ฌ์ฉ
iris.loc[:,'Sepal Length']
# ํ์ด์ฌ ๋ด์ฅ ํจ์ ์ฌ์ฉ
iris['Sepal Length']
## 0 5.1
## 1 4.9
## 2 4.7
## 3 4.6
## 4 5.0
## ...
## 145 6.7
## 146 6.3
## 147 6.5
## 148 6.2
## 149 5.9
## Name: Sepal Length, Length: 150, dtype: float64
6. ์กฐ๊ฑด์ ๋ง๋ ๋ฐ์ดํฐ ํํฐ๋งํ๊ธฐ
'Species'๊ฐ 'Iris-setosa'์ธ ํ๋ค๋ง ์ ํํ์ฌ ์๋ก์ด ๋ฐ์ดํฐํ๋ ์์ ๋ง๋์ธ์.
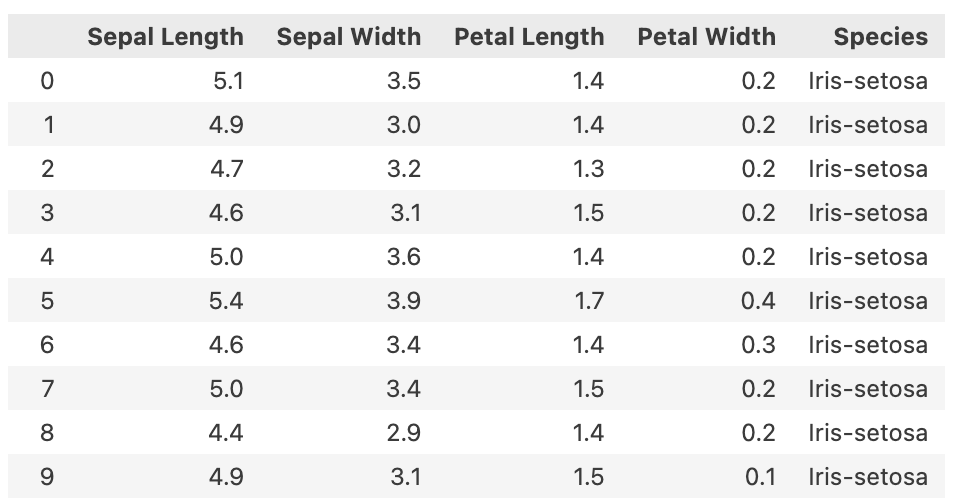
df = iris[iris['Species']=='Iris-setosa']
df
- ๋ถ๋ฆฌ์ธ ์ธ๋ฑ์ฑ์ ํ์ฉํ๊ธฐ
- ์กฐ๊ฑด(iris์ species ์นผ๋ผ์์ 'iris-setosa'๊ฐ์ ๊ฐ์ง๋ ํ๋ค๋ง ์ ํํด๋ผ)
โ`iris['Species']=='Iris-setosa'` - iris[์กฐ๊ฑด] : ์กฐ๊ฑด์ ๋ง๋ ํ์ iris ๋ฐ์ดํฐ์์ ์ ํ
- ์กฐ๊ฑด(iris์ species ์นผ๋ผ์์ 'iris-setosa'๊ฐ์ ๊ฐ์ง๋ ํ๋ค๋ง ์ ํํด๋ผ)
7. ๊ทธ๋ฃน๋ณ ํต๊ณ๋ ๊ณ์ฐํ๊ธฐ
๊ฐ ํ์ข ('Species')๋ณ๋ก 'Sepal Length'์ ํ๊ท ์ ๊ณ์ฐํ์ธ์.
iris.groupby('Species')['Sepal Length'].mean()
## Species
## Iris-setosa 5.006
## Iris-versicolor 5.936
## Iris-virginica 6.588
## Name: Sepal Length, dtype: float64
- groupby & ์ง๊ณํจ์ ์ฌ์ฉํด ๊ทธ๋ฃน๋ณ ํต๊ณ๋ ๊ณ์ฐ
8. ์๋ก์ด ์ด ์ถ๊ฐํ๊ธฐ
๊ฐ ํ์ 'Sepal Length'์ 'Sepal Width'์ ํฉ์ ๊ณ์ฐํ์ฌ ์๋ก์ด ์ด 'Sepal Sum'์ ์ถ๊ฐํ์ธ์.
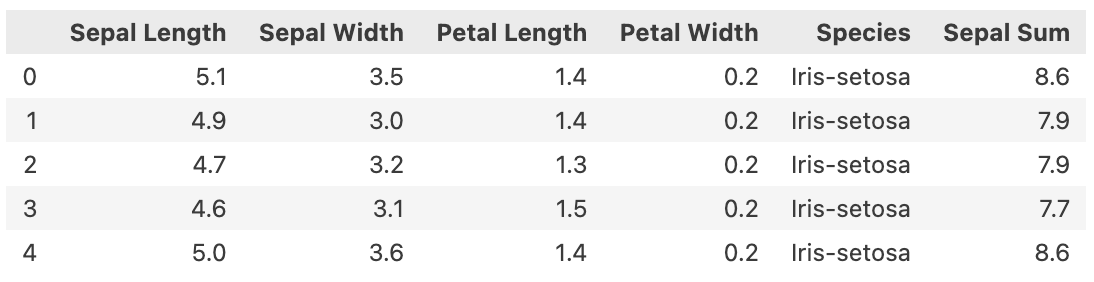
iris['Sepal Sum'] = iris['Sepal Length']+iris['Sepal Width']
- ์๋ก์ด ์นผ๋ผ์ ์ถ๊ฐํ๋ ๋ฐฉ๋ฒ
- dataframe['์๋ก์ด ์นผ๋ผ ๋ช '] = ์นผ๋ผ ์์ ๋ค์ด๊ฐ ๊ฐ
9. ๋ฐ์ดํฐ ์ ๋ ฌํ๊ธฐ
'Petal Length' ๊ธฐ์ค์ผ๋ก ๋ฐ์ดํฐํ๋ ์์ ๋ด๋ฆผ์ฐจ์์ผ๋ก ์ ๋ ฌํ์ธ์.
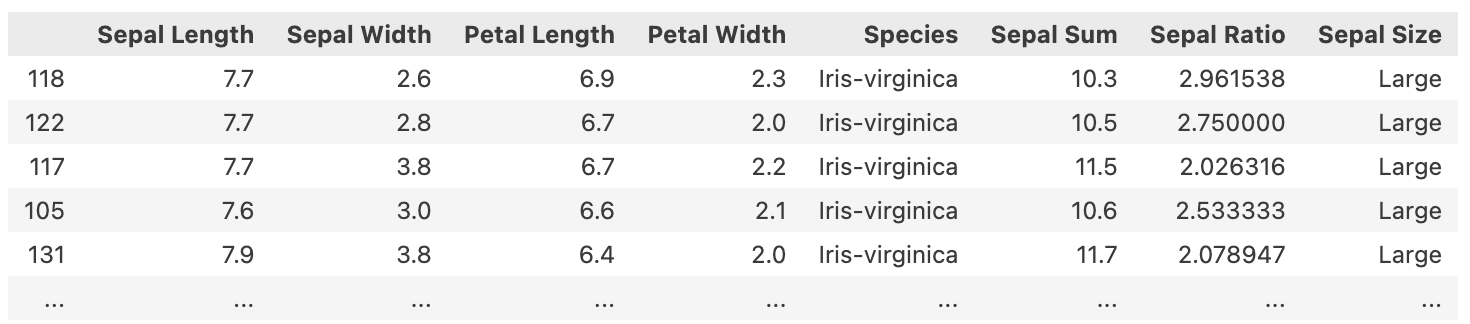
iris.sort_values('Petal Length', ascending = False)
- ๋ฐ์ดํฐ ํ๋ ์์์ ํน์ ์นผ๋ผ์ ๊ฐ์ ๊ธฐ์ค์ผ๋ก sorting ํ๋ ํจ์ โ sort_values('์ ๋ ฌ ๊ธฐ์ค ์ปฌ๋ผ')
10. ํน์ ๊ฐ ์ธ๊ธฐ
๊ฐ ํ์ข ('Species')๋ณ๋ก ๋ช ๊ฐ์ ์ํ์ด ์๋์ง ์ธ์ด๋ณด์ธ์.
iris.value_counts('Species')
## Species
## Iris-setosa 50
## Iris-versicolor 50
## Iris-virginica 50
## Name: count, dtype: int64
- ํน์ ์ปฌ๋ผ์ ๊ฐ์๋ฅผ ์ธ์ด์ฃผ๋ ํจ์ โ value_counts('์ปฌ๋ผ๋ช ')
์ฑ๋ฆฐ์ง ๋ฌธํญ
11. ๋ฐ์ดํฐ ๊ฒฐํฉํ๊ธฐ
'Sepal Length'์ 'Petal Length'์ ํ๊ท ์ ๊ณ์ฐํ ํ, ์ด๋ฅผ ์๋ก์ด ๋ฐ์ดํฐํ๋ ์์ผ๋ก ๊ฒฐํฉํ์ธ์.
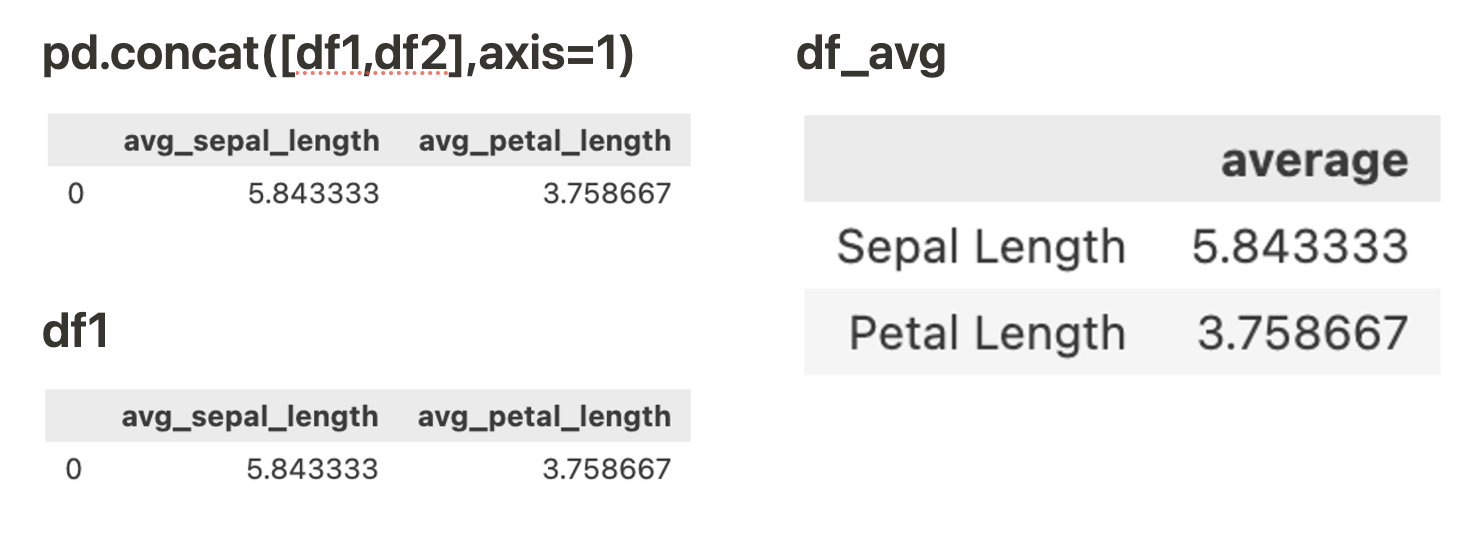
# 1 Concat ์ฌ์ฉ
df1 = pd.DataFrame({'avg_sepal_length' : [iris['Sepal Length'].mean()]})
df2 = pd.DataFrame({'avg_petal_length' : [iris['Petal Length'].mean()]})
pd.concat([df1,df2],axis=1)
# 2 ๋์
๋๋ฆฌ ์ด์ฉํด ๋ฐ์ดํฐ ํ๋ ์ ๋ง๋ค๊ธฐ
df1 = pd.DataFrame({'avg_sepal_length' : [iris['Sepal Length'].mean()],
'avg_petal_length' : [iris['Petal Length'].mean()]})
# 3 ํ๋ฒ์ ๋ฐ์ดํฐ ํ๋ ์์ผ๋ก ๋ฌถ๊ธฐ
df_avg = pd.DataFrame(iris[['Sepal Length','Petal Length']].mean())
df_avg.columns = ['average']
12. ํผ๋ฒํ ์ด๋ธ ๋ง๋ค๊ธฐ
๊ฐ ํ์ข ๋ณ 'Sepal Length'์ 'Petal Length'์ ํ๊ท ์ ํผ๋ฒ ํ ์ด๋ธ๋ก ๋ง๋ค์ด ๋ณด์ธ์.
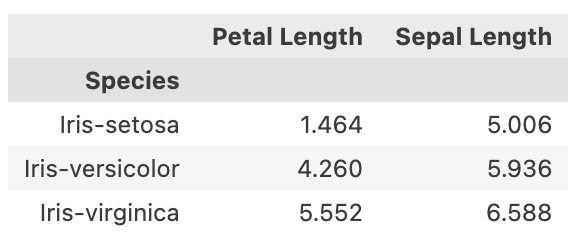
iris.pivot_table(index = 'Species', values = ['Sepal Length', 'Petal Length'], aggfunc = 'mean')
13. ๊ฒฐ์ธก๊ฐ ์ฒ๋ฆฌ
'Sepal Width' ์ด์ ์์๋ก ๊ฒฐ์ธก๊ฐ์ 10๊ฐ ์ถ๊ฐํ๊ณ , ๊ฒฐ์ธก๊ฐ์ ์ฒ๋ฆฌํ๋ ๋ฐฉ๋ฒ์ ๋ ๊ฐ์ง ์ด์ ์ ์ฉํด ๋ณด์ธ์.
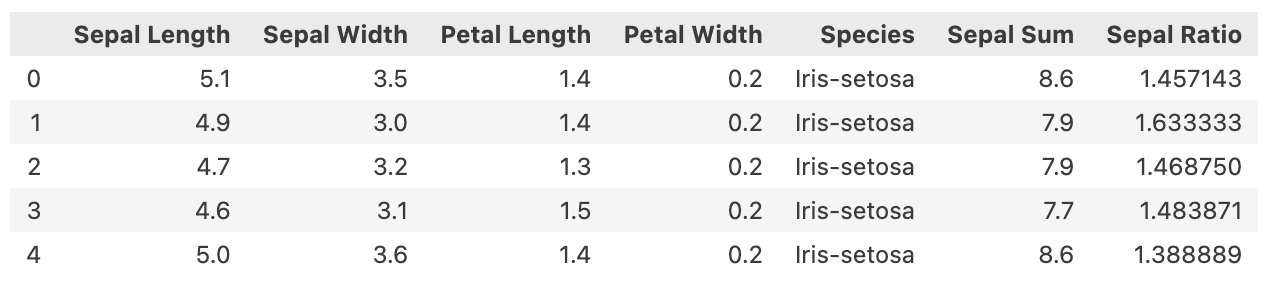
# ์์๋ก ๊ฒฐ์ธก๊ฐ ์ถ๊ฐํ๊ธฐ
import numpy as np
# ์์๋ก ๊ฒฐ์ธก๊ฐ 10๊ฐ ์ถ๊ฐ
iris_with_nan = iris.copy()
iris_with_nan.loc[np.random.choice(iris_with_nan.index, 10), 'Sepal Width'] = np.nan
iris_with_nan.head()
print(f'๊ฒฐ์ธก์น๋ฅผ ์ฒ๋ฆฌํ๊ธฐ ์ ๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ {iris_with_nan.isna().sum().sum()}๊ฐ์
๋๋ค.')
# (1) ๊ฒฐ์ธก๊ฐ ๋ ๋ฆฌ๊ธฐ
dropall = iris_with_nan.dropna(how='any')
print(f'๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ {dropall.isna().sum().sum()}๊ฐ์
๋๋ค.')
# (2) ๋์ฒด๊ฐ์ ์ฑ์๋ฃ๊ธฐ : Species ์ปฌ๋ผ์ ํ๊ท ๊ฐ์ผ๋ก ๊ฒฐ์ธก์น๋ฅผ ์ฑ์ฐ๊ธฐ
fill_data = iris_with_nan['Sepal Length'].mean()
print(f"Species ์ปฌ๋ผ์ ํ๊ท ๊ฐ์ {fill_data}์
๋๋ค.")
fill_avg = iris_with_nan.fillna(fill_data)
print(f'๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ {fill_avg.isna().sum().sum()}๊ฐ์
๋๋ค.')
## ๊ฒฐ์ธก์น๋ฅผ ์ฒ๋ฆฌํ๊ธฐ ์ ๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ 10๊ฐ์
๋๋ค.
## ๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ 0๊ฐ์
๋๋ค.
## Species ์ปฌ๋ผ์ ํ๊ท ๊ฐ์ 5.843333333333334์
๋๋ค.
## ๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ 0๊ฐ์
๋๋ค.
14. ๋ฐ์ดํฐ ๋ณํ
'Sepal Length'์ 'Sepal Width'์ ๋น์จ์ ๊ณ์ฐํ์ฌ ์๋ก์ด ์ด 'Sepal Ratio'๋ฅผ ์ถ๊ฐํ์ธ์.
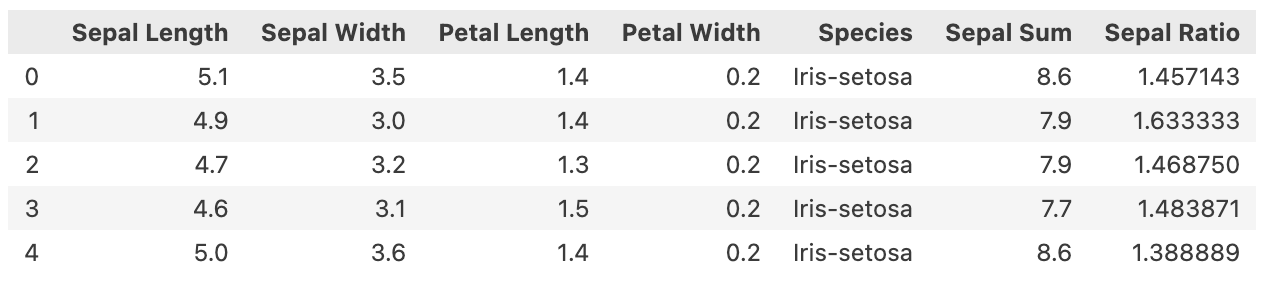
iris['Sepal Ratio'] = iris['Sepal Length']/iris['Sepal Width']
15. ํน์ ์กฐ๊ฑด์ ๋ฐ๋ฅธ ์๋ก์ด ์ด ์์ฑ
'Sepal Length'๊ฐ 5.0 ์ด์์ธ ๊ฒฝ์ฐ 'Large', ๋ฏธ๋ง์ธ ๊ฒฝ์ฐ 'Small'์ ๊ฐ์ผ๋ก ๊ฐ์ง๋ ์๋ก์ด ์ด 'Sepal Size'๋ฅผ ์์ฑํ์ธ์.
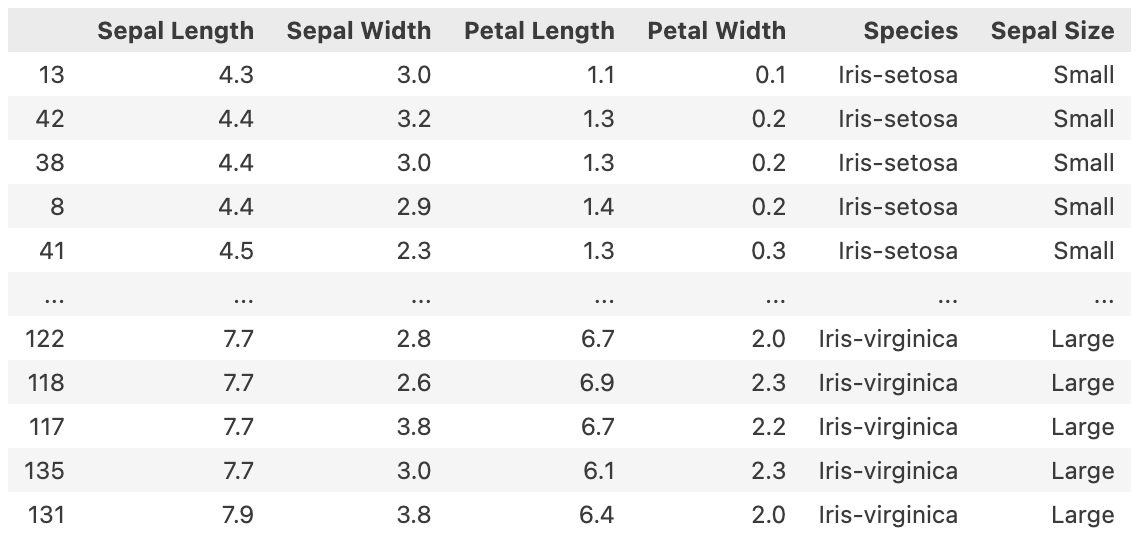
# 1. ๋ฐ๋ณต๋ฌธ ์ด์ฉํ๊ธฐ(๋ฆฌ์คํธ ์ปดํ๋ฆฌํจ์
)
iris['Sepal Size'] = ['Large' if i>=5 else 'Small' for i in iris['Sepal Length']]
# 3. loc ์ด์ฉํ๊ธฐ
iris.loc[(iris['Sepal Length']>=5),'Sepal Size'] ='Large'
iris.loc[(iris['Sepal Length']<5),'Sepal Size'] ='Small'
๐ก ๋ฆฌ์คํธ ์ปดํ๋ฆฌํธ์ ์ด ์๋ ๋ฐ๋ณต๋ฌธ์ ์ด์ฉํ๋ ๊ฒ์ด ๋ถ๊ฐ๋ฅํ๋ค!iris.sort_values('Sepal Length')
for i in iris['Sepal Length']: if i>= 5: iris['Sepal Size'] = 'Large' else: iris['Sepal Size'] = 'Small'โ
์์ ๊ฐ์ด ๋ฐ๋ณต๋ฌธ์ ์์ฑ ํ๋ฉด ๋ ๊ฒ ๊ฐ์ง๋ง, sort values๋ฅผ ํด๋ณด๋ฉด ์์ ์ด๋ฏธ์ง์ ๊ฐ์ด sepal size๊ฐ ์ ์ ํ์ง ์๊ฒ ๋ค์ด๊ฐ ๊ฒ์ ํ์ธํ ์ ์๋ค. ํํฐ๋ ๋ง์์ผ๋ก๋ ํ๋ค์ค์ ๋ก์ง์ ์ด๋ฌํ ๊ฒ์ ํฌํจํ์ง ์๋ ๊ฒ ๊ฐ๋ค๊ณ ..!!! ๋๋๋ก์ด๋ฉด loc๋ ๋ฆฌ์คํธ ์ปดํ๋ฆฌํจ์ ์ ์ฌ์ฉํ๋ ๊ฒ์ด ์ข๊ฒ ๋ค.
16. ๋ค์ํ ํต๊ณ๋ ๊ณ์ฐ
๊ฐ ํ์ข (Species)๋ณ๋ก 'Sepal Length'์ 'Sepal Width'์ ํฉ๊ณ, ํ๊ท , ํ์คํธ์ฐจ๋ฅผ ๊ณ์ฐํ์ธ์.
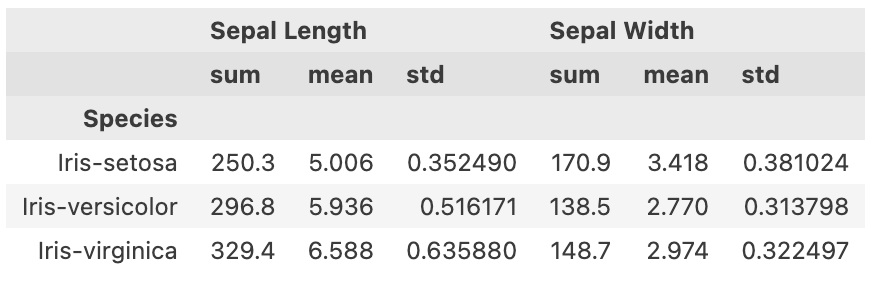
iris.groupby('Species')[['Sepal Length', 'Sepal Width']].agg(['sum', 'mean', 'std'])
17. ๋ณต์กํ ์กฐ๊ฑด ํํฐ๋ง
'Sepal Length'๊ฐ 5.0 ์ด์์ด๊ณ 'Sepal Width'๊ฐ 3.5 ์ดํ์ธ ๋ฐ์ดํฐ๋ง ์ ํํ๊ณ , ์ด ๋ฐ์ดํฐ์ 'Petal Length'์ 'Petal Width'์ ํฉ์ ์๋ก์ด ์ด 'Petal Sum'์ผ๋ก ์ถ๊ฐํ์ธ์.
new_iris = iris.loc[(iris['Sepal Length']>= 5)&(iris['Sepal Width']<=3.5)]
new_iris['Petal Sum'] = new_iris['Petal Length']+ new_iris['Petal Width']
18. ์ฐ์ ๋ ๊ทธ๋ฆฌ๊ธฐ
'Sepal Length'์ 'Sepal Width'์ ๊ด๊ณ๋ฅผ ๋ํ๋ด๋ ์ฐ์ ๋๋ฅผ ๊ทธ๋ฆฌ์ธ์. ๊ฐ ํ์ข ๋ณ๋ก ๋ค๋ฅธ ์์์ ์ฌ์ฉํ์ธ์.
- Matplotlib ver.
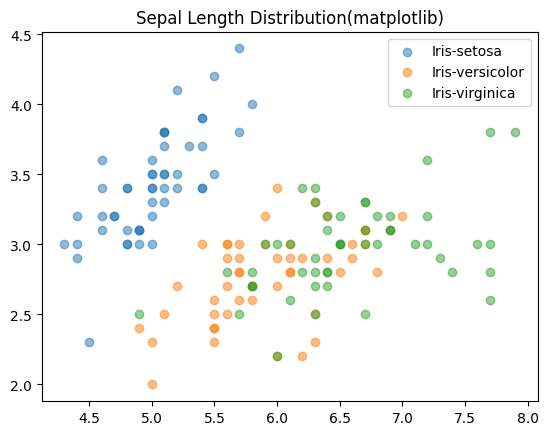
for s in iris['Species'].unique():
plt.scatter(iris[iris['Species']==s]['Sepal Length'],
iris[iris['Species']==s]['Sepal Width'],
label = s, alpha = 0.5)
plt.legend(iris['Species'].unique())
plt.title('Sepal Length Distribution(matplotlib)')
- Seaborn ver.
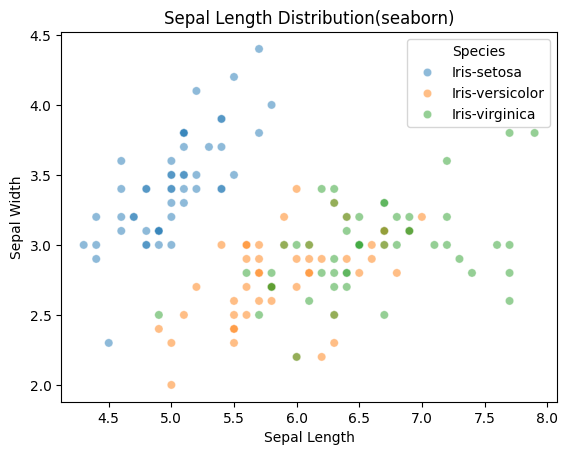
sns.scatterplot(data=iris, x='Sepal Length', y = 'Sepal Width', hue='Species', alpha = 0.5)
19. ํ์คํ ๊ทธ๋จ ๊ทธ๋ฆฌ๊ธฐ
'Sepal Length'์ ๋ถํฌ๋ฅผ ๋ํ๋ด๋ ํ์คํ ๊ทธ๋จ์ ๊ทธ๋ฆฌ์ธ์. ๊ฐ ํ์ข ๋ณ๋ก ๋ค๋ฅธ ์์์ ์ฌ์ฉํ์ธ์.
- Matplotlib ver.
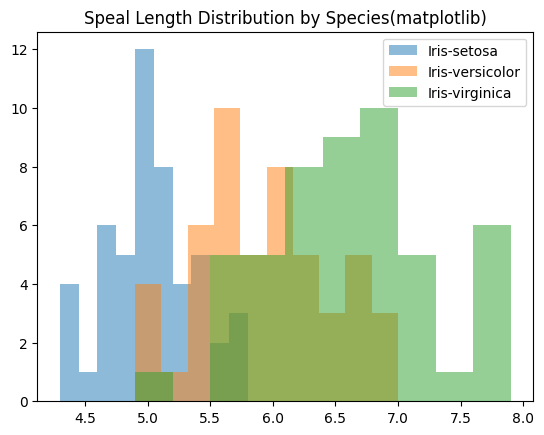
for s in iris['Species'].unique():
plt.hist(iris[iris['Species']==s]['Sepal Length'], label= iris['Species'].unique(), alpha = 0.5)
plt.legend(iris['Species'].unique())
plt.title('Speal Length Distribution by Species(matplotlib)')
- Seaborn ver.
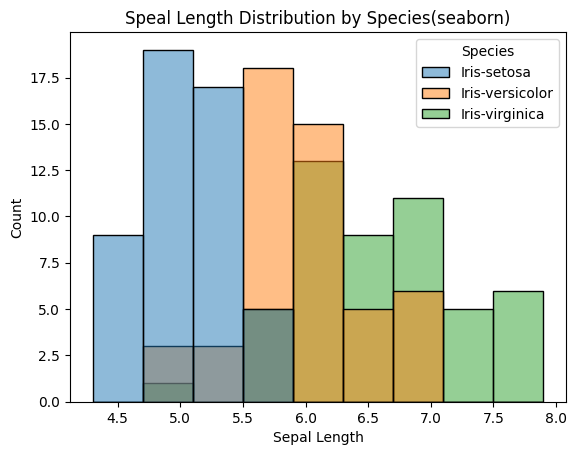
sns.histplot(data=iris, x='Sepal Length', hue='Species', alpha=0.5)
plt.title('Speal Length Distribution by Species(seaborn)')
20. ๋ฐ์คํ๋กฏ ๊ทธ๋ฆฌ๊ธฐ
๊ฐ ํ์ข ๋ณ๋ก 'Petal Length'์ ๋ถํฌ๋ฅผ ๋ํ๋ด๋ ๋ฐ์คํ๋กฏ์ ๊ทธ๋ฆฌ์ธ์.
- Matplotlib ver.
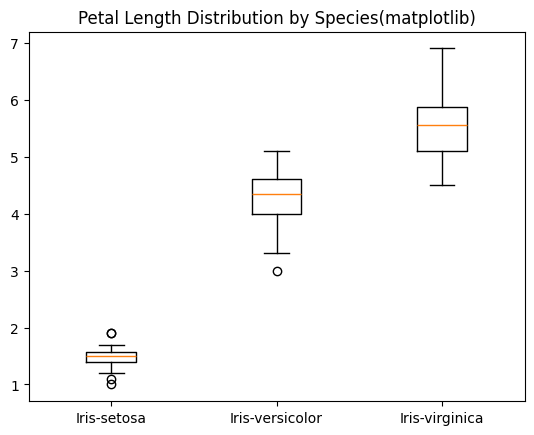
plt.boxplot([iris[iris['Species']==s]['Petal Length'] for s in iris['Species'].unique()],
labels = iris['Species'].unique())
plt.title('Petal Length Distribution by Species(matplotlib)')
- Seaborn ver.
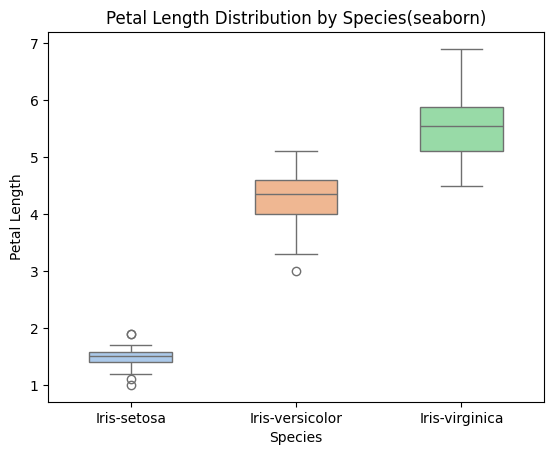
sns.boxplot(data=iris, x='Species', y='Petal Length', palette='pastel', width=0.5)
plt.title('Petal Length Distribution by Species(seaborn)')
'๐ Today I Learn > ๐ Python' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
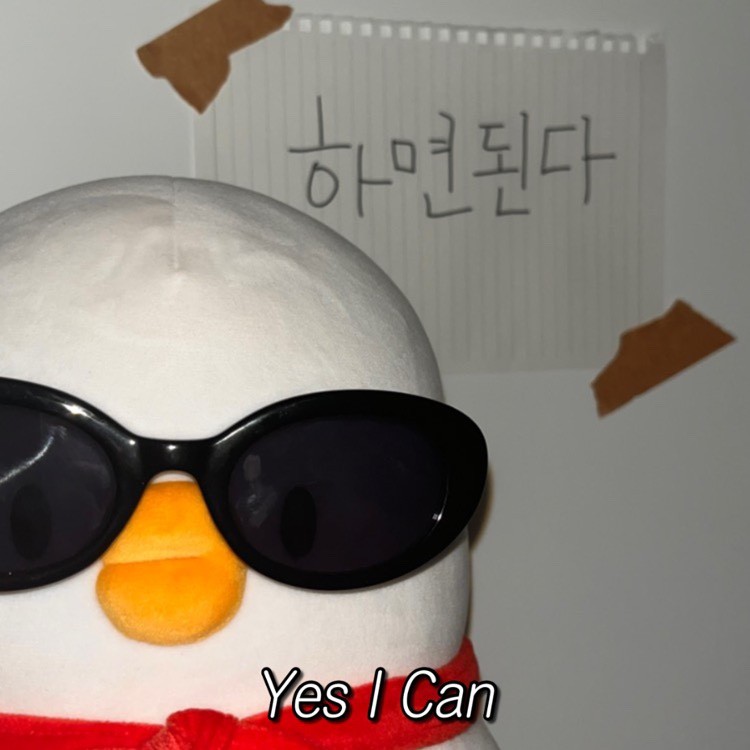
๋ผ์ด๋ธ๋ฌ๋ฆฌ ๋ถ๋ฌ์ค๊ธฐ
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
๋ฒ ์ด์ง ๋ฌธํญ
1. ๋ฐ์ดํฐ ๋ถ๋ฌ์ค๊ธฐ
pandas๋ฅผ importํ ๋ค์ ๋ฐ์ดํฐ๋ฅผ ๋ถ๋ฌ์์ ๋ฐ์ดํฐ๋ฅผ ํ์ธํ์ธ์.
# ๋ฐ์ดํฐ ๋ถ๋ฌ์ค๊ธฐ
url = 'https://archive.ics.uci.edu/ml/machine-learning-databases/iris/iris.data'
columns = ['Sepal Length', 'Sepal Width', 'Petal Length', 'Petal Width', 'Species']
iris = pd.read_csv(url, header=None, names=columns)
2. ๋ฐ์ดํฐ ๊ตฌ์กฐ ํ์ ํ๊ธฐ
๋ฐ์ดํฐ์ ์ ์ฒซ 5ํ์ ์ถ๋ ฅํ๊ณ , ๋ฐ์ดํฐ์ ๊ตฌ์กฐ๋ฅผ ํ์ ํ์ธ์.
iris.head()
- ๋ฐ์ดํฐํ๋ ์์์ ์ฒ์ 5๊ฐ์ ํ์ ์ถ๋ ฅํ๋ ํจ์ โ head()
3. ๋ฐ์ดํฐ ์์ฝ์ ๋ณด ํ์ธํ๊ธฐ
๋ฐ์ดํฐ์ ์ ์์ฝ ์ ๋ณด๋ฅผ ํ์ธํ์ธ์.
iris.info()
## <class 'pandas.core.frame.DataFrame'>
## RangeIndex: 150 entries, 0 to 149
## Data columns (total 5 columns):
## # Column Non-Null Count Dtype
## --- ------ -------------- -----
## 0 Sepal Length 150 non-null float64
## 1 Sepal Width 150 non-null float64
## 2 Petal Length 150 non-null float64
## 3 Petal Width 150 non-null float64
## 4 Species 150 non-null object
## dtypes: float64(4), object(1)
## memory usage: 6.0+ KB
- ๋ฐ์ดํฐ์ ์ ์์ฝ ์ ๋ณด๋ฅผ ํ์ธํ๋ ํจ์ โ info()
4. ๊ธฐ์ด ํต๊ณ๋ ํ์ธํ๊ธฐ
๊ฐ ์ด์ ๊ธฐ์ด ํต๊ณ๋(ํ๊ท , ํ์คํธ์ฐจ, ์ต์๊ฐ, ์ต๋๊ฐ ๋ฑ)์ ์ถ๋ ฅํ์ธ์.
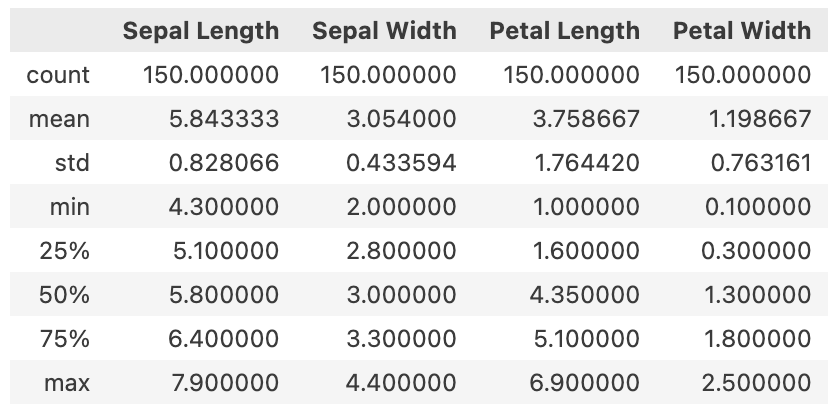
iris.describe()
- ๊ธฐ์ดํต๊ณ๋์ ์ถ๋ ฅํ๋ ํจ์ โ describe()
5. ํน์ ์ด ์ ํํ๊ธฐ
'Sepal Length' ์ด๋ง ์ ํํ์ฌ ์ถ๋ ฅํ์ธ์.
# ํ๋ค์ค ํจ์ ์ฌ์ฉ
iris.loc[:,'Sepal Length']
# ํ์ด์ฌ ๋ด์ฅ ํจ์ ์ฌ์ฉ
iris['Sepal Length']
## 0 5.1
## 1 4.9
## 2 4.7
## 3 4.6
## 4 5.0
## ...
## 145 6.7
## 146 6.3
## 147 6.5
## 148 6.2
## 149 5.9
## Name: Sepal Length, Length: 150, dtype: float64
6. ์กฐ๊ฑด์ ๋ง๋ ๋ฐ์ดํฐ ํํฐ๋งํ๊ธฐ
'Species'๊ฐ 'Iris-setosa'์ธ ํ๋ค๋ง ์ ํํ์ฌ ์๋ก์ด ๋ฐ์ดํฐํ๋ ์์ ๋ง๋์ธ์.
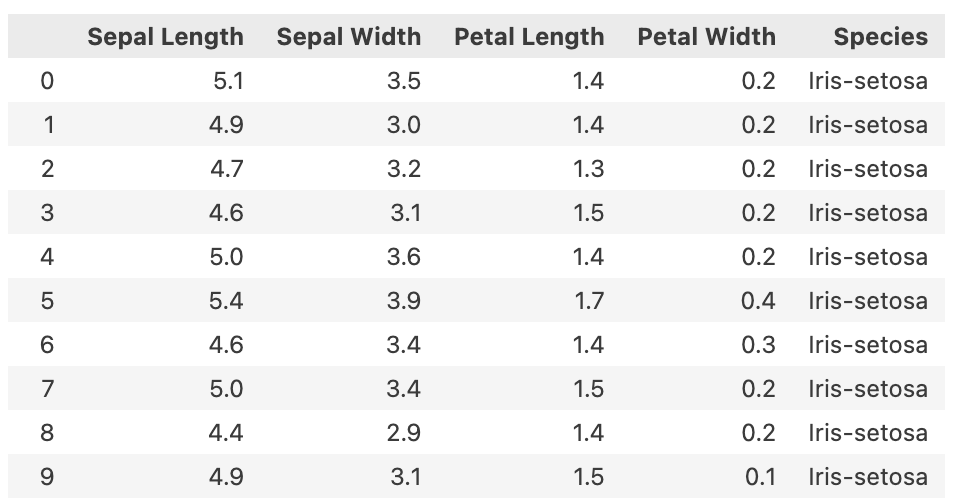
df = iris[iris['Species']=='Iris-setosa']
df
- ๋ถ๋ฆฌ์ธ ์ธ๋ฑ์ฑ์ ํ์ฉํ๊ธฐ
- ์กฐ๊ฑด(iris์ species ์นผ๋ผ์์ 'iris-setosa'๊ฐ์ ๊ฐ์ง๋ ํ๋ค๋ง ์ ํํด๋ผ)
โ`iris['Species']=='Iris-setosa'` - iris[์กฐ๊ฑด] : ์กฐ๊ฑด์ ๋ง๋ ํ์ iris ๋ฐ์ดํฐ์์ ์ ํ
- ์กฐ๊ฑด(iris์ species ์นผ๋ผ์์ 'iris-setosa'๊ฐ์ ๊ฐ์ง๋ ํ๋ค๋ง ์ ํํด๋ผ)
7. ๊ทธ๋ฃน๋ณ ํต๊ณ๋ ๊ณ์ฐํ๊ธฐ
๊ฐ ํ์ข ('Species')๋ณ๋ก 'Sepal Length'์ ํ๊ท ์ ๊ณ์ฐํ์ธ์.
iris.groupby('Species')['Sepal Length'].mean()
## Species
## Iris-setosa 5.006
## Iris-versicolor 5.936
## Iris-virginica 6.588
## Name: Sepal Length, dtype: float64
- groupby & ์ง๊ณํจ์ ์ฌ์ฉํด ๊ทธ๋ฃน๋ณ ํต๊ณ๋ ๊ณ์ฐ
8. ์๋ก์ด ์ด ์ถ๊ฐํ๊ธฐ
๊ฐ ํ์ 'Sepal Length'์ 'Sepal Width'์ ํฉ์ ๊ณ์ฐํ์ฌ ์๋ก์ด ์ด 'Sepal Sum'์ ์ถ๊ฐํ์ธ์.
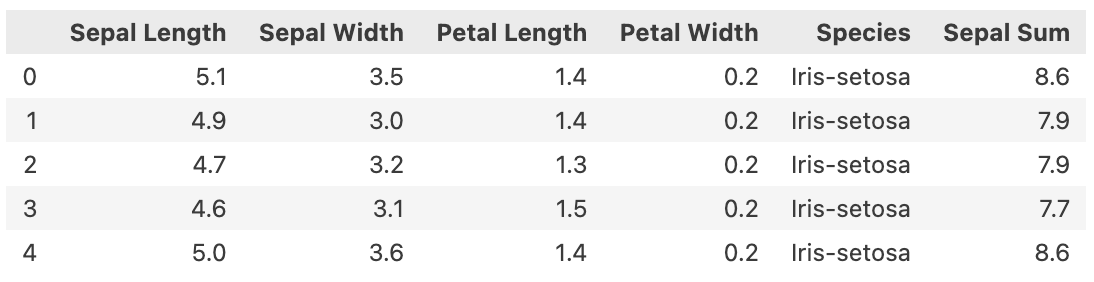
iris['Sepal Sum'] = iris['Sepal Length']+iris['Sepal Width']
- ์๋ก์ด ์นผ๋ผ์ ์ถ๊ฐํ๋ ๋ฐฉ๋ฒ
- dataframe['์๋ก์ด ์นผ๋ผ ๋ช '] = ์นผ๋ผ ์์ ๋ค์ด๊ฐ ๊ฐ
9. ๋ฐ์ดํฐ ์ ๋ ฌํ๊ธฐ
'Petal Length' ๊ธฐ์ค์ผ๋ก ๋ฐ์ดํฐํ๋ ์์ ๋ด๋ฆผ์ฐจ์์ผ๋ก ์ ๋ ฌํ์ธ์.
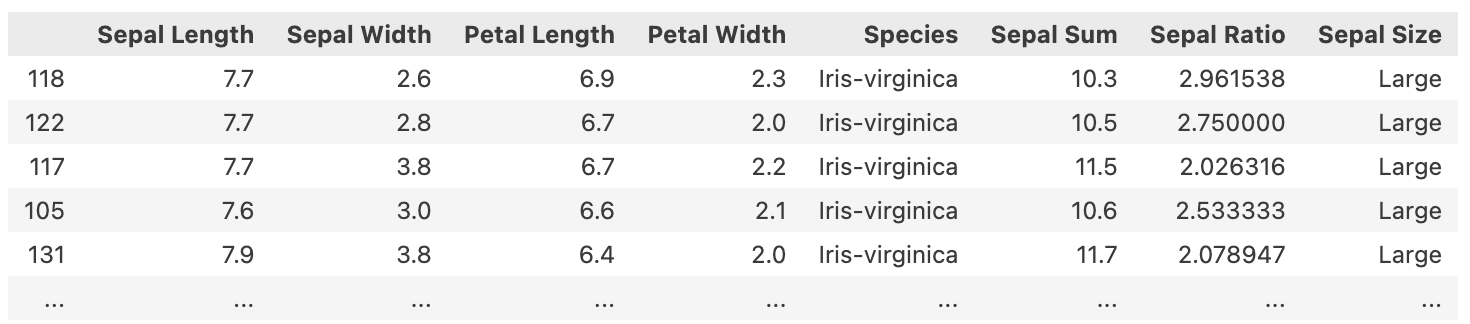
iris.sort_values('Petal Length', ascending = False)
- ๋ฐ์ดํฐ ํ๋ ์์์ ํน์ ์นผ๋ผ์ ๊ฐ์ ๊ธฐ์ค์ผ๋ก sorting ํ๋ ํจ์ โ sort_values('์ ๋ ฌ ๊ธฐ์ค ์ปฌ๋ผ')
10. ํน์ ๊ฐ ์ธ๊ธฐ
๊ฐ ํ์ข ('Species')๋ณ๋ก ๋ช ๊ฐ์ ์ํ์ด ์๋์ง ์ธ์ด๋ณด์ธ์.
iris.value_counts('Species')
## Species
## Iris-setosa 50
## Iris-versicolor 50
## Iris-virginica 50
## Name: count, dtype: int64
- ํน์ ์ปฌ๋ผ์ ๊ฐ์๋ฅผ ์ธ์ด์ฃผ๋ ํจ์ โ value_counts('์ปฌ๋ผ๋ช ')
์ฑ๋ฆฐ์ง ๋ฌธํญ
11. ๋ฐ์ดํฐ ๊ฒฐํฉํ๊ธฐ
'Sepal Length'์ 'Petal Length'์ ํ๊ท ์ ๊ณ์ฐํ ํ, ์ด๋ฅผ ์๋ก์ด ๋ฐ์ดํฐํ๋ ์์ผ๋ก ๊ฒฐํฉํ์ธ์.
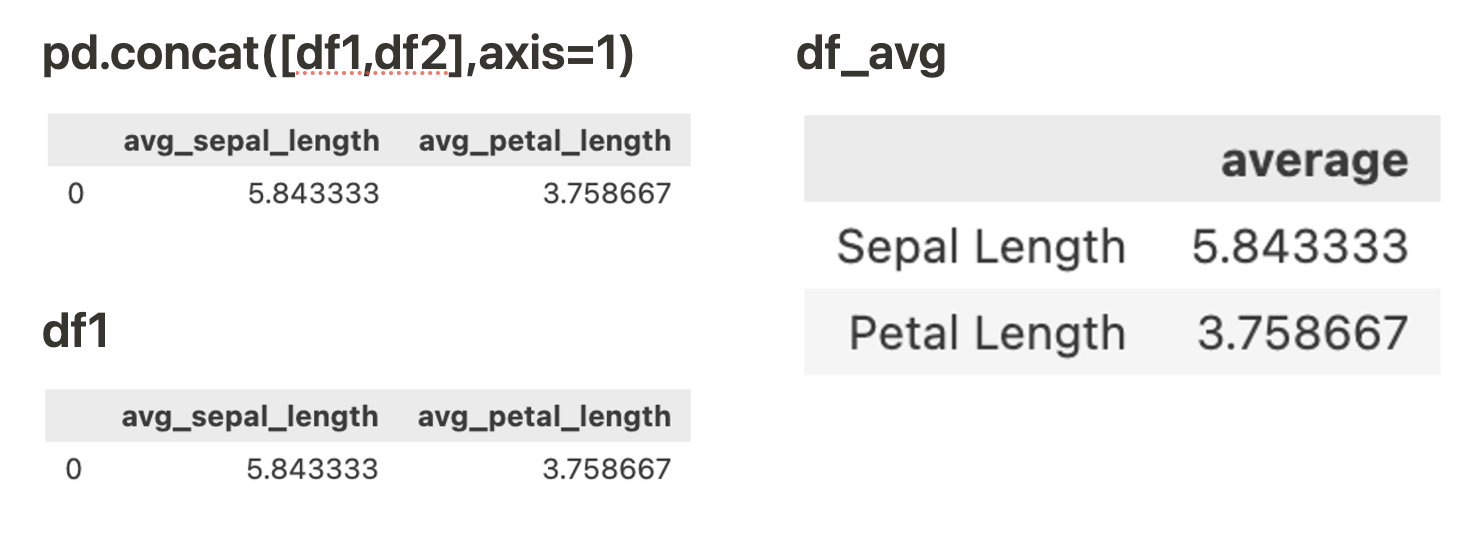
# 1 Concat ์ฌ์ฉ
df1 = pd.DataFrame({'avg_sepal_length' : [iris['Sepal Length'].mean()]})
df2 = pd.DataFrame({'avg_petal_length' : [iris['Petal Length'].mean()]})
pd.concat([df1,df2],axis=1)
# 2 ๋์
๋๋ฆฌ ์ด์ฉํด ๋ฐ์ดํฐ ํ๋ ์ ๋ง๋ค๊ธฐ
df1 = pd.DataFrame({'avg_sepal_length' : [iris['Sepal Length'].mean()],
'avg_petal_length' : [iris['Petal Length'].mean()]})
# 3 ํ๋ฒ์ ๋ฐ์ดํฐ ํ๋ ์์ผ๋ก ๋ฌถ๊ธฐ
df_avg = pd.DataFrame(iris[['Sepal Length','Petal Length']].mean())
df_avg.columns = ['average']
12. ํผ๋ฒํ ์ด๋ธ ๋ง๋ค๊ธฐ
๊ฐ ํ์ข ๋ณ 'Sepal Length'์ 'Petal Length'์ ํ๊ท ์ ํผ๋ฒ ํ ์ด๋ธ๋ก ๋ง๋ค์ด ๋ณด์ธ์.
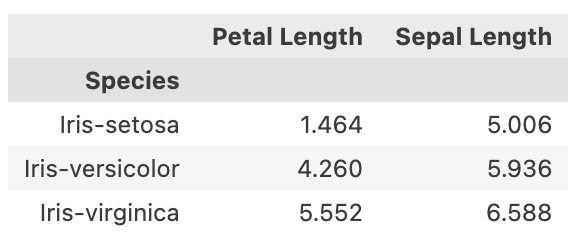
iris.pivot_table(index = 'Species', values = ['Sepal Length', 'Petal Length'], aggfunc = 'mean')
13. ๊ฒฐ์ธก๊ฐ ์ฒ๋ฆฌ
'Sepal Width' ์ด์ ์์๋ก ๊ฒฐ์ธก๊ฐ์ 10๊ฐ ์ถ๊ฐํ๊ณ , ๊ฒฐ์ธก๊ฐ์ ์ฒ๋ฆฌํ๋ ๋ฐฉ๋ฒ์ ๋ ๊ฐ์ง ์ด์ ์ ์ฉํด ๋ณด์ธ์.
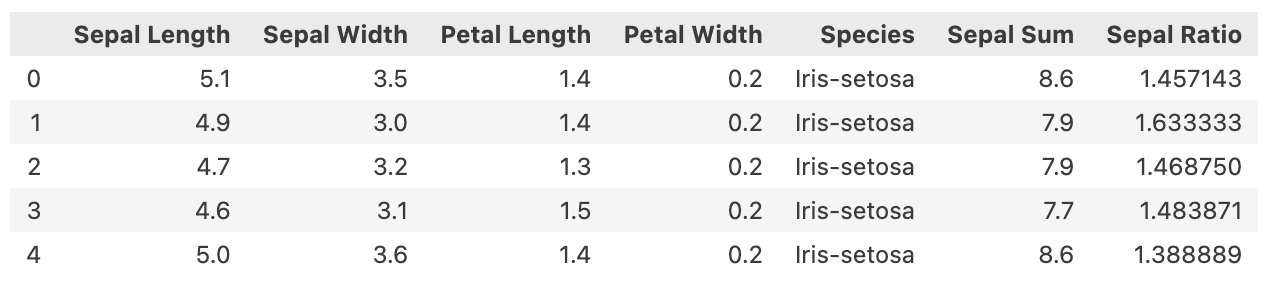
# ์์๋ก ๊ฒฐ์ธก๊ฐ ์ถ๊ฐํ๊ธฐ
import numpy as np
# ์์๋ก ๊ฒฐ์ธก๊ฐ 10๊ฐ ์ถ๊ฐ
iris_with_nan = iris.copy()
iris_with_nan.loc[np.random.choice(iris_with_nan.index, 10), 'Sepal Width'] = np.nan
iris_with_nan.head()
print(f'๊ฒฐ์ธก์น๋ฅผ ์ฒ๋ฆฌํ๊ธฐ ์ ๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ {iris_with_nan.isna().sum().sum()}๊ฐ์
๋๋ค.')
# (1) ๊ฒฐ์ธก๊ฐ ๋ ๋ฆฌ๊ธฐ
dropall = iris_with_nan.dropna(how='any')
print(f'๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ {dropall.isna().sum().sum()}๊ฐ์
๋๋ค.')
# (2) ๋์ฒด๊ฐ์ ์ฑ์๋ฃ๊ธฐ : Species ์ปฌ๋ผ์ ํ๊ท ๊ฐ์ผ๋ก ๊ฒฐ์ธก์น๋ฅผ ์ฑ์ฐ๊ธฐ
fill_data = iris_with_nan['Sepal Length'].mean()
print(f"Species ์ปฌ๋ผ์ ํ๊ท ๊ฐ์ {fill_data}์
๋๋ค.")
fill_avg = iris_with_nan.fillna(fill_data)
print(f'๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ {fill_avg.isna().sum().sum()}๊ฐ์
๋๋ค.')
## ๊ฒฐ์ธก์น๋ฅผ ์ฒ๋ฆฌํ๊ธฐ ์ ๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ 10๊ฐ์
๋๋ค.
## ๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ 0๊ฐ์
๋๋ค.
## Species ์ปฌ๋ผ์ ํ๊ท ๊ฐ์ 5.843333333333334์
๋๋ค.
## ๊ฒฐ์ธก๊ฐ์ ๊ฐ์๋ 0๊ฐ์
๋๋ค.
14. ๋ฐ์ดํฐ ๋ณํ
'Sepal Length'์ 'Sepal Width'์ ๋น์จ์ ๊ณ์ฐํ์ฌ ์๋ก์ด ์ด 'Sepal Ratio'๋ฅผ ์ถ๊ฐํ์ธ์.
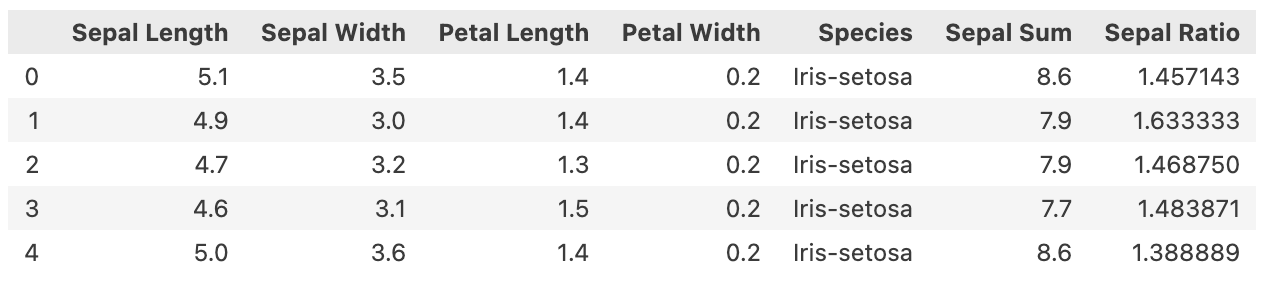
iris['Sepal Ratio'] = iris['Sepal Length']/iris['Sepal Width']
15. ํน์ ์กฐ๊ฑด์ ๋ฐ๋ฅธ ์๋ก์ด ์ด ์์ฑ
'Sepal Length'๊ฐ 5.0 ์ด์์ธ ๊ฒฝ์ฐ 'Large', ๋ฏธ๋ง์ธ ๊ฒฝ์ฐ 'Small'์ ๊ฐ์ผ๋ก ๊ฐ์ง๋ ์๋ก์ด ์ด 'Sepal Size'๋ฅผ ์์ฑํ์ธ์.
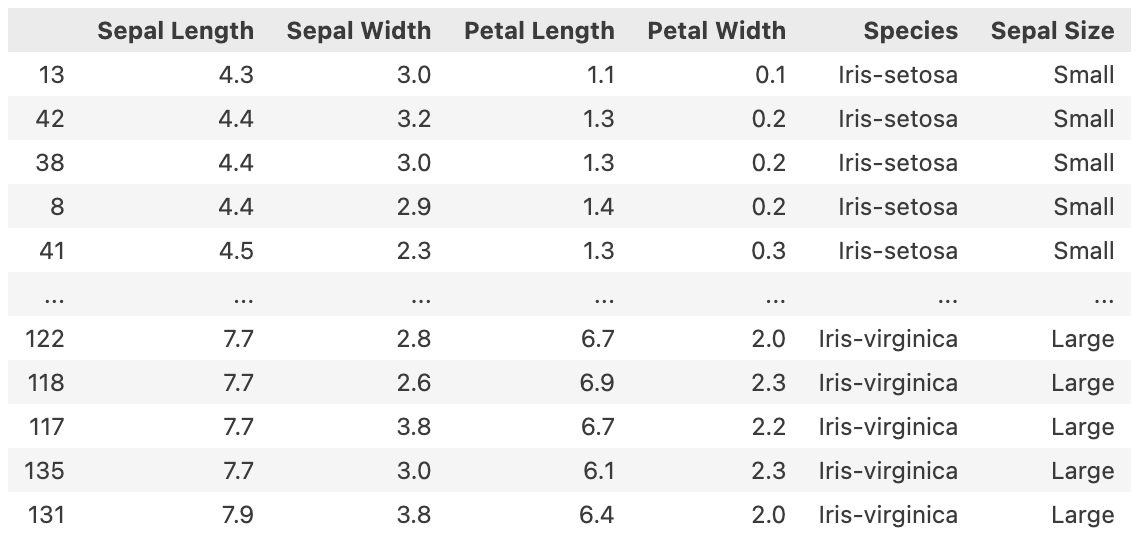
# 1. ๋ฐ๋ณต๋ฌธ ์ด์ฉํ๊ธฐ(๋ฆฌ์คํธ ์ปดํ๋ฆฌํจ์
)
iris['Sepal Size'] = ['Large' if i>=5 else 'Small' for i in iris['Sepal Length']]
# 3. loc ์ด์ฉํ๊ธฐ
iris.loc[(iris['Sepal Length']>=5),'Sepal Size'] ='Large'
iris.loc[(iris['Sepal Length']<5),'Sepal Size'] ='Small'
๐ก ๋ฆฌ์คํธ ์ปดํ๋ฆฌํธ์ ์ด ์๋ ๋ฐ๋ณต๋ฌธ์ ์ด์ฉํ๋ ๊ฒ์ด ๋ถ๊ฐ๋ฅํ๋ค!iris.sort_values('Sepal Length')
for i in iris['Sepal Length']: if i>= 5: iris['Sepal Size'] = 'Large' else: iris['Sepal Size'] = 'Small'โ
์์ ๊ฐ์ด ๋ฐ๋ณต๋ฌธ์ ์์ฑ ํ๋ฉด ๋ ๊ฒ ๊ฐ์ง๋ง, sort values๋ฅผ ํด๋ณด๋ฉด ์์ ์ด๋ฏธ์ง์ ๊ฐ์ด sepal size๊ฐ ์ ์ ํ์ง ์๊ฒ ๋ค์ด๊ฐ ๊ฒ์ ํ์ธํ ์ ์๋ค. ํํฐ๋ ๋ง์์ผ๋ก๋ ํ๋ค์ค์ ๋ก์ง์ ์ด๋ฌํ ๊ฒ์ ํฌํจํ์ง ์๋ ๊ฒ ๊ฐ๋ค๊ณ ..!!! ๋๋๋ก์ด๋ฉด loc๋ ๋ฆฌ์คํธ ์ปดํ๋ฆฌํจ์ ์ ์ฌ์ฉํ๋ ๊ฒ์ด ์ข๊ฒ ๋ค.
16. ๋ค์ํ ํต๊ณ๋ ๊ณ์ฐ
๊ฐ ํ์ข (Species)๋ณ๋ก 'Sepal Length'์ 'Sepal Width'์ ํฉ๊ณ, ํ๊ท , ํ์คํธ์ฐจ๋ฅผ ๊ณ์ฐํ์ธ์.
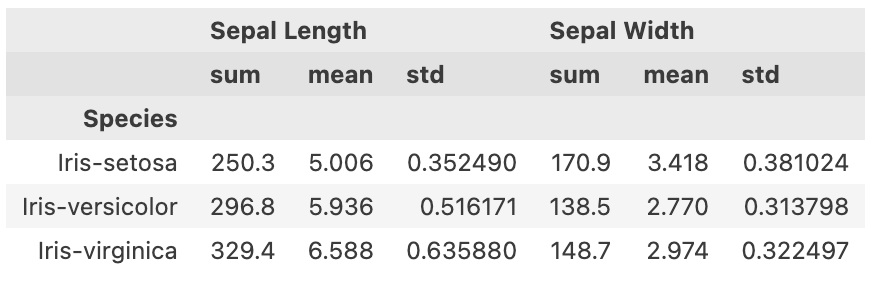
iris.groupby('Species')[['Sepal Length', 'Sepal Width']].agg(['sum', 'mean', 'std'])
17. ๋ณต์กํ ์กฐ๊ฑด ํํฐ๋ง
'Sepal Length'๊ฐ 5.0 ์ด์์ด๊ณ 'Sepal Width'๊ฐ 3.5 ์ดํ์ธ ๋ฐ์ดํฐ๋ง ์ ํํ๊ณ , ์ด ๋ฐ์ดํฐ์ 'Petal Length'์ 'Petal Width'์ ํฉ์ ์๋ก์ด ์ด 'Petal Sum'์ผ๋ก ์ถ๊ฐํ์ธ์.
new_iris = iris.loc[(iris['Sepal Length']>= 5)&(iris['Sepal Width']<=3.5)]
new_iris['Petal Sum'] = new_iris['Petal Length']+ new_iris['Petal Width']
18. ์ฐ์ ๋ ๊ทธ๋ฆฌ๊ธฐ
'Sepal Length'์ 'Sepal Width'์ ๊ด๊ณ๋ฅผ ๋ํ๋ด๋ ์ฐ์ ๋๋ฅผ ๊ทธ๋ฆฌ์ธ์. ๊ฐ ํ์ข ๋ณ๋ก ๋ค๋ฅธ ์์์ ์ฌ์ฉํ์ธ์.
- Matplotlib ver.
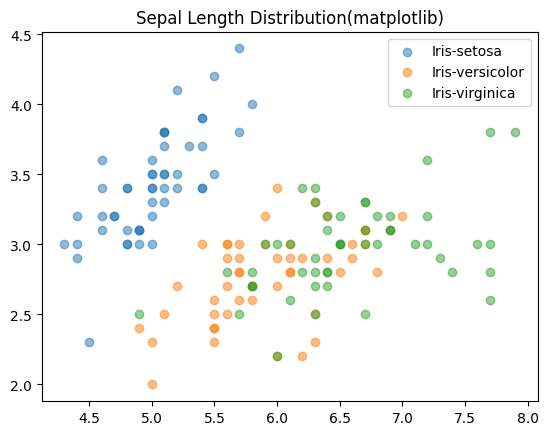
for s in iris['Species'].unique():
plt.scatter(iris[iris['Species']==s]['Sepal Length'],
iris[iris['Species']==s]['Sepal Width'],
label = s, alpha = 0.5)
plt.legend(iris['Species'].unique())
plt.title('Sepal Length Distribution(matplotlib)')
- Seaborn ver.
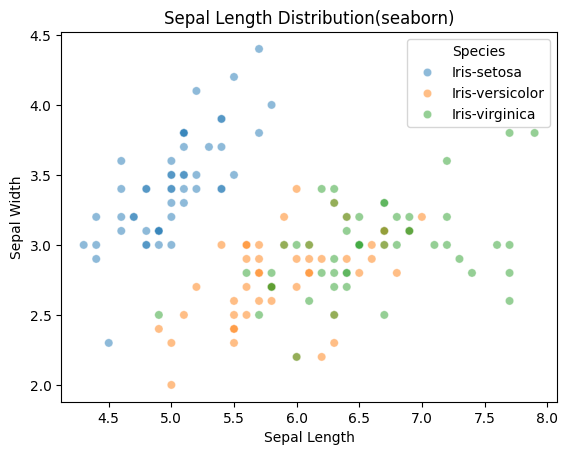
sns.scatterplot(data=iris, x='Sepal Length', y = 'Sepal Width', hue='Species', alpha = 0.5)
19. ํ์คํ ๊ทธ๋จ ๊ทธ๋ฆฌ๊ธฐ
'Sepal Length'์ ๋ถํฌ๋ฅผ ๋ํ๋ด๋ ํ์คํ ๊ทธ๋จ์ ๊ทธ๋ฆฌ์ธ์. ๊ฐ ํ์ข ๋ณ๋ก ๋ค๋ฅธ ์์์ ์ฌ์ฉํ์ธ์.
- Matplotlib ver.
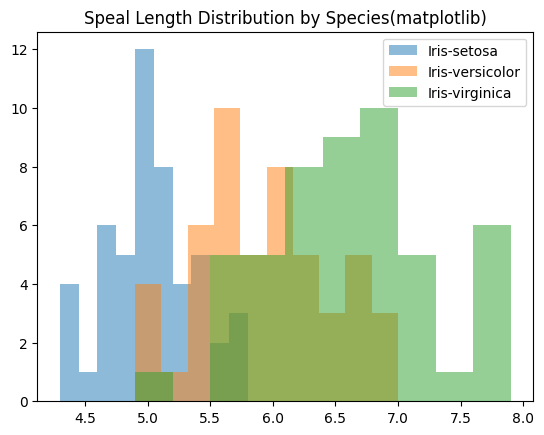
for s in iris['Species'].unique():
plt.hist(iris[iris['Species']==s]['Sepal Length'], label= iris['Species'].unique(), alpha = 0.5)
plt.legend(iris['Species'].unique())
plt.title('Speal Length Distribution by Species(matplotlib)')
- Seaborn ver.
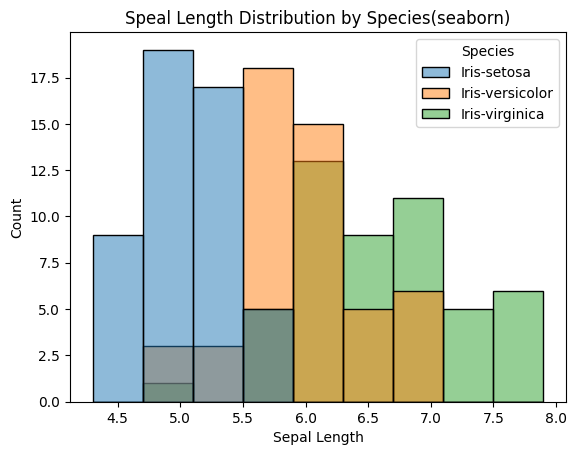
sns.histplot(data=iris, x='Sepal Length', hue='Species', alpha=0.5)
plt.title('Speal Length Distribution by Species(seaborn)')
20. ๋ฐ์คํ๋กฏ ๊ทธ๋ฆฌ๊ธฐ
๊ฐ ํ์ข ๋ณ๋ก 'Petal Length'์ ๋ถํฌ๋ฅผ ๋ํ๋ด๋ ๋ฐ์คํ๋กฏ์ ๊ทธ๋ฆฌ์ธ์.
- Matplotlib ver.
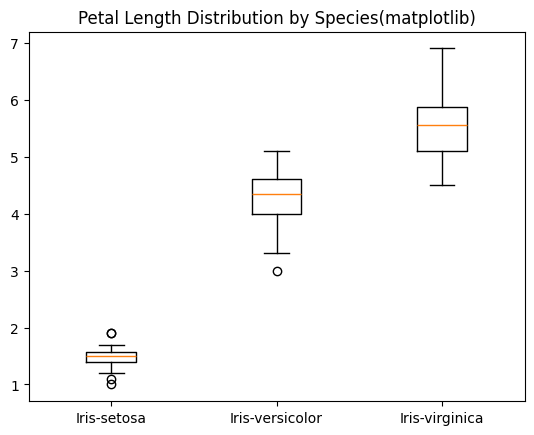
plt.boxplot([iris[iris['Species']==s]['Petal Length'] for s in iris['Species'].unique()],
labels = iris['Species'].unique())
plt.title('Petal Length Distribution by Species(matplotlib)')
- Seaborn ver.
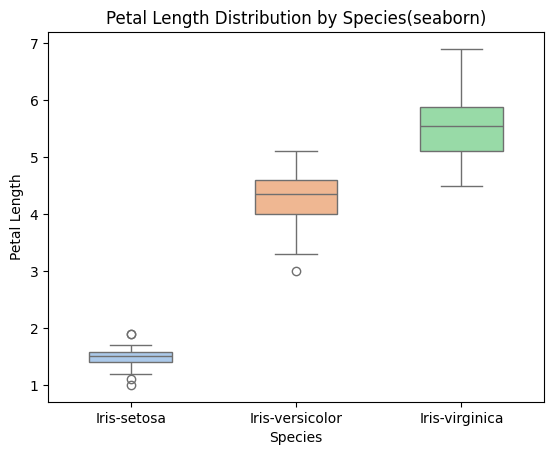
sns.boxplot(data=iris, x='Species', y='Petal Length', palette='pastel', width=0.5)
plt.title('Petal Length Distribution by Species(seaborn)')